You cant create web server on VPS in 5 minutes. This article will show you how to do so.
Create a new VPS with Debian 10 Operation system. Connect to it via ssh and the run the folowing commands.
apt update apt upgrade
Open a new file in your favorite editor. The simplest is nano.
nano web-server.py
Copy-paste (or type) the following code. Do not forget to replace <your_public_ip> with IP of your VPS.
#!/usr/bin/python3 from http.server import BaseHTTPRequestHandler, HTTPServer import time hostName = "<your_public_ip>" serverPort = 80 class MyServer(BaseHTTPRequestHandler): def do_GET(self): self.send_response(200) self.send_header("Content-type", "text/html") self.end_headers() self.wfile.write(bytes("OK", "utf-8")) if __name__ == "__main__": webServer = HTTPServer((hostName, serverPort), MyServer) print("Server started http://%s:%s" % (hostName, serverPort)) try: webServer.serve_forever() except KeyboardInterrupt: pass webServer.server_close() print("Server stopped.")
Ctrl+o – to save the file. Ctrl+x – to exit nano. Change mode to executable:
chmod a+x web-server.py
Run the server. (If you bind to first 1024 ports then your need run service as a sudo).
./web-server.py
Now you can open browser, enter <your_public_ip> and enjoy the result 😎.
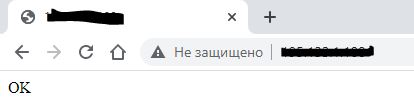
Customize Web Server port
If you need another port than you edit serverPort variable. If port is 38800 than you do not need sudo to run the server.
#!/usr/bin/python3 from http.server import BaseHTTPRequestHandler, HTTPServer import time hostName = "<your_public_ip>" serverPort = 38800
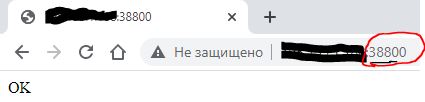
Customize page path
class MyServer(BaseHTTPRequestHandler): def do_GET(self): self.send_response(200) self.send_header("Content-type", "text/html") self.end_headers() if self.path == "/": self.wfile.write(bytes("Main page", "utf-8")) return if self.path == "/page1": self.wfile.write(bytes("page1", "utf-8")) return if self.path == "/page2": self.wfile.write(bytes("page2", "utf-8")) return
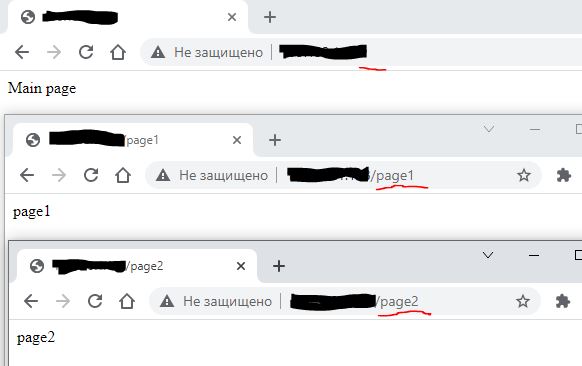
Response with JSON
#!/usr/bin/python3 from http.server import BaseHTTPRequestHandler, HTTPServer import time import json hostName = "<your_public_ip>" serverPort = 80 class MyServer(BaseHTTPRequestHandler): def do_GET(self): self.send_response(200) self.send_header("Content-type", "application/json") self.end_headers() response = { "id": 12, "fieldStr": "text", "version": "1.2.3.4" } self.wfile.write(bytes(json.dumps(response), "utf-8"))
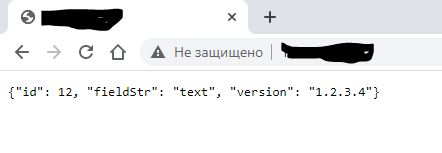
Conclusion
Creating Web server in 5 minutes is very useful feature for testing.